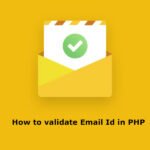
email_validation
Email validation is a crucial step in web development, ensuring that user input is accurate and formatted correctly. This process helps prevent errors in data submission and reduces the chances of malicious attacks through invalid email addresses. In this guide, we’ll explore various methods to perform email validation in PHP, offering you practical examples and best practices.
Why Is Email Validation Important?
Validating email addresses is essential for several reasons:
- Data Integrity: Ensures that the data collected is accurate, helping maintain a clean database.
- User Experience: By preventing users from entering invalid emails, you enhance their experience and reduce frustration.
- Spam Reduction: Reduces the risk of spam and abuse, protecting your application and users.
- Communication: Valid emails ensure that your communication reaches the intended recipients.
Methods for Email Validation in PHP
There are multiple approaches to validate email addresses in PHP. Let’s discuss some of the most common methods.
1. Using the filter_var()
Function
The simplest and most efficient way to validate an email address in PHP is by using the filter_var()
function. This built-in function filters a variable with a specified filter.
Here’s how you can use it:
$email = "example@example.com";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo “The email address ‘$email‘ is considered valid.”;
} else {
echo “The email address ‘$email‘ is considered invalid.”;
}
This method checks the format of the email and returns true
if it is valid and false
if it is not.
2. Regular Expressions
Another way to validate an email address is by using regular expressions. Regular expressions provide a flexible way to match specific patterns in strings.
Here’s a sample code snippet:
$email = "example@example.com";
$pattern = "/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/";
if (preg_match($pattern, $email)) {echo “The email address ‘$email‘ is valid.”;
} else {
echo “The email address ‘$email‘ is invalid.”;
}
This method allows for more control over what constitutes a valid email address. However, it requires a good understanding of regular expressions.
3. Using checkdnsrr()
Function
To further enhance the validation process, you can check whether the domain of the email address actually exists. This is done using the checkdnsrr()
function, which checks DNS records for a given domain.
Here’s how it works:
$email = "example@example.com";
list($user, $domain) = explode(‘@’, $email);
if (filter_var($email, FILTER_VALIDATE_EMAIL) && checkdnsrr($domain)) {
echo “The email address ‘$email‘ is valid and the domain exists.”;
} else {
echo “The email address ‘$email‘ is invalid or the domain does not exist.”;
}
This method ensures not only that the email format is correct but also that the domain can receive emails.
Best Practices for Email Validation in PHP
- Sanitize Input: Always sanitize user input to prevent SQL injection and XSS attacks.
- User Feedback: Provide clear and immediate feedback to users on invalid email submissions.
- Limit Attempts: Implement a limit on the number of attempts a user can make to input an email address to reduce spam.
Implementing Email Validation in a Form
To give you a practical example, let’s integrate email validation into a simple HTML form using PHP.
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Email Validation Form</title>
</head>
<body>
<form method="POST" action="">
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" value="Submit">
</form>
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo “The email address ‘$email’ is valid.”;
} else {
echo “The email address ‘$email’ is invalid.”;
}
}
?>
</body>
</html>
This form collects an email address and validates it upon submission, providing feedback to the user.
Common Errors in Email Validation
While validating email addresses, developers often encounter a few common issues:
- Too Strict Validation: Overly strict regex patterns can reject valid emails.
- Ignoring Domain Existence: Only validating the format may lead to accepting non-existent domains.
- User Experience: Not providing clear messages can lead to user frustration.
Considering International Email Formats
As globalization increases, so does the need to accommodate international email formats in your validation processes. Many email addresses may contain non-ASCII characters, especially in domains. The filter_var()
function in PHP is equipped to handle these cases, but you may need to implement additional checks to ensure compatibility with various character sets. Utilizing libraries like intl
can enhance your validation by allowing you to handle internationalized email addresses correctly. Moreover, understanding the nuances of different formats can improve user experience for a global audience, ensuring that your application is accessible and user-friendly to people from diverse linguistic backgrounds.
Conclusion
In conclusion, validating email addresses is an essential aspect of web development that ensures data integrity, enhances user experience, and prevents spam. By using methods like filter_var()
, regular expressions, and checkdnsrr()
, you can implement effective email validation in PHP. Remember to keep user experience in mind and provide clear feedback to improve the overall interaction with your application.